A manual memory managed,
compiled to C99,
programming language with off-side rule syntax,
and YakshaLisp sublanguage.
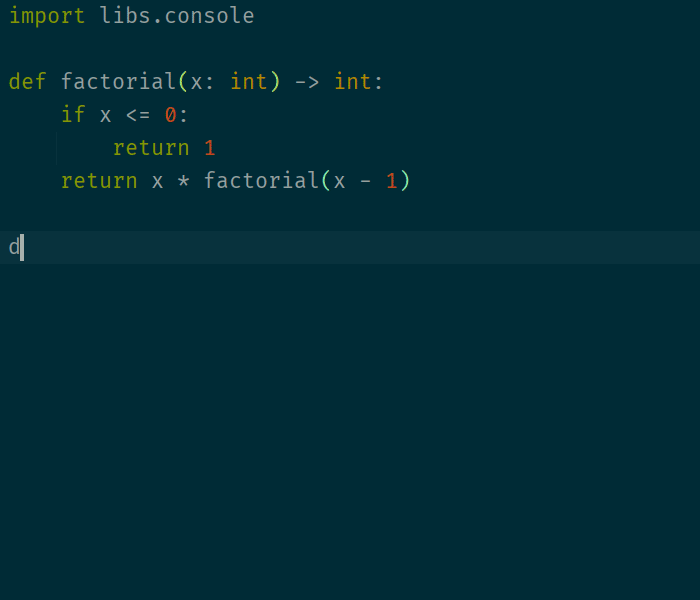
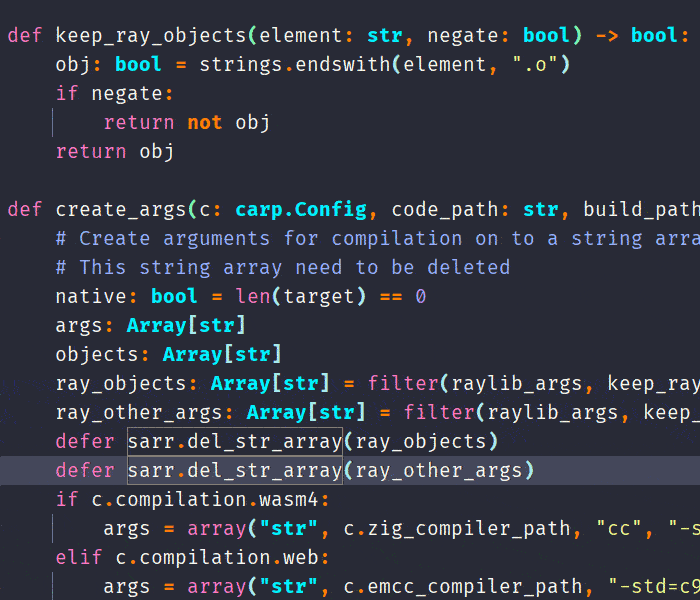
Simple. Strict. Speedy.
Language syntax is minimalistic and has similarities to Python.
Manual memory management is required.
Use YakshaLisp to create macros (Hygenic, Non-hygenic, or non-hygenic metamacros).
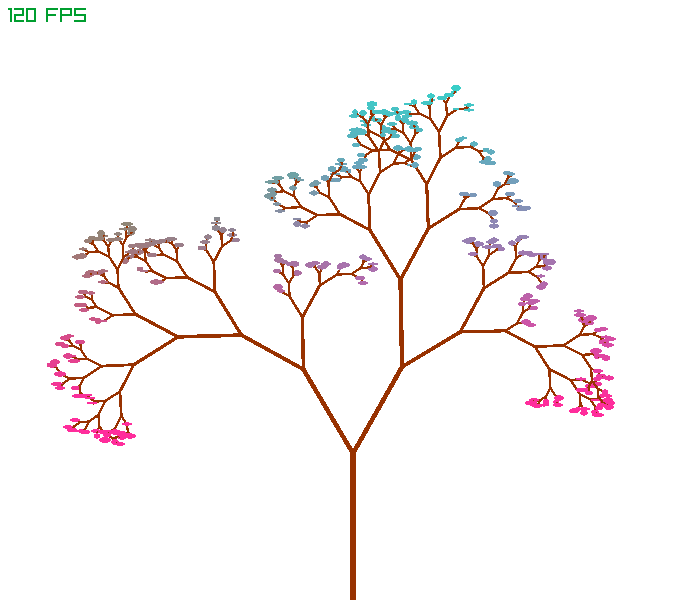
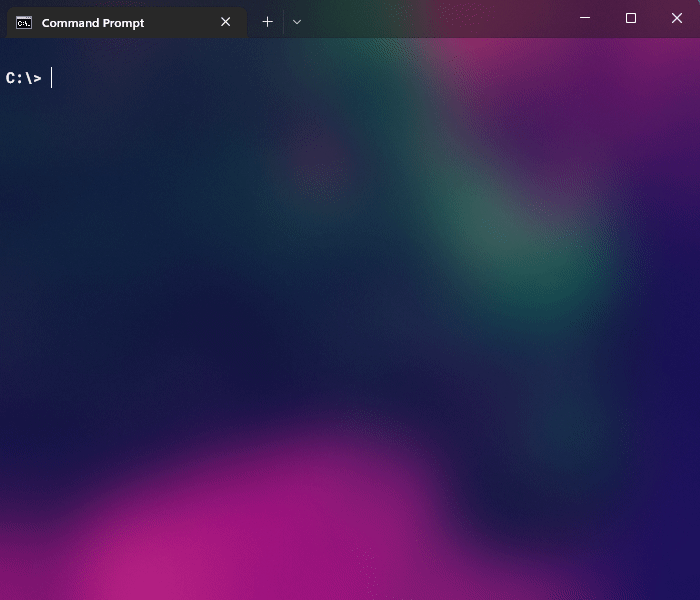
A versatile new tool
Yaksha aims to be a simple general purpose language with a minimalistic standard library.
One goal is to have easy access to GPU/parallel programming (this has not yet begun, and hopefully we can get there) using OpenCL.
There will be first class support for Windows.
At the moment, the author plans on using the language (once it reaches a usable level) in personal projects.