This section needs rewriting
This describes Yaksha language grammar.
Lot learned from Crafting Interpreters
book and Python grammar page.
Statements
in-progress
This section describes statements and program structure. Program is made from one or more program statements. Standard statements are what goes inside functions.
program: import_statement* program_declaration*
program_declarations:
| const_statement
| struct_statement
| def_statement
standard_statement:
| compound_statement
| simple_statement
compound_statement:
| if_statement
| while_statement
simple_statement:
| let_statement
| return_statement
| defer_statement
| "pass"
| "break"
| "continue"
| expr_statement
| del_statement
| c_code_statement
# Because defer can use del statement or any another expression.
# Del statement has two components with and without statement end.
base_del_statement: "del" expr
del_statement: base_del_statement st_end
# Defer can use a del statement or an expr
defer_statement: "defer" (base_del_statement | expr) st_end
# Functions
def_statement: annotation* "def" name "(" parameters? ")" block st_end
# Let statement creates variables
let_statement: name ":" data_type ("=" expr)? st_end
# If statement
if_statement: "if" block ("else" block)?
# While statement
while_statement: "while" block
# Custom data structures
# Struct value data types must be present
struct_value: name ":" data_type st_end
struct_block: ":" newline ba_indent struct_value+ ba_dedent
stuct_statement: annotation* "class" name struct_block
# Expression statements, calling functions etc.
expr_statement: expr st_end
# Return statement
return_statement: "return" expr st_end
# Constants compiles to #defines.
const_statement: "const" name "=" (number | string)
# Import statements include other source files and perform name mangling
# You need to always rename level 2+ imports
import_statement: "import" name ( ("." name)+ "as" name )? st_end
# Dumps unmodified C code. It would be better to encapsulate these.
# When used with @native functions compiles to a c macro
c_code_statement: "ccode" (string | triple_quote_string) st_end
# Multiline or single simple statement blocks
block: ":" (newline ba_indent standard_statement+ ba_dedent | simple_statement)
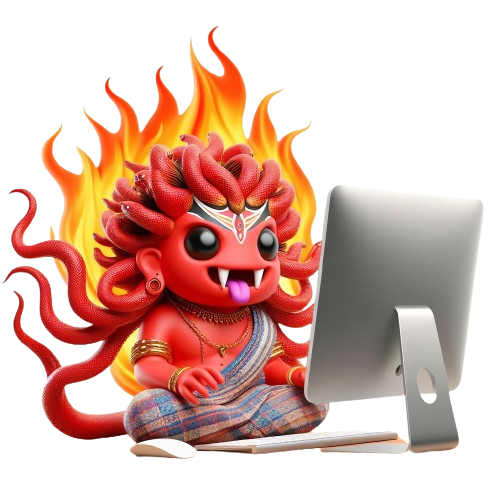
defer
statement is special, it can take either adel
statement or any expression.- This allows you to defer a
del
. - Example -
defer del my_element
Data Type
data_type: data_type_part | name ("." name)? ("[" data_type_args "]")?
data_type_args: data_type ("," data_type)*
data_type_part: name | primitive_data_type
primitive_data_type: "int" | "i8" | "i16" | "i32" | "i64" |
"u8" | "u16" | "u32" | "u64" | "str" | "float" | "bool" | "None" |
"f32" | "f64"
Data type describes, primitives, custom data types and non primitives.
Expressions
Expressions are itsy bitsy pieces that make up parts of statements.
expr: assignment
assignment: (fncall ".")? name "=" assignment
| logical_not;
logical_not: "not" logic_or
logic_or: logic_and ("or" logic_and)*
logic_and: equality ("and" equality)*
equality: comparision (("!=" | "==") comparision)*
comparision: term ((">" | ">=" | "<" | "<=") term)*
term: factor (("-" | "+") factor)*
factor: unary (("/" | "*") unary)*
unary: "-" unary | fncall
fncall: primary ( "(" arguments? ")" | "[" expr "]" | "." name )*
primary: "True" | "False" | "None" | number | string | triple_quote_string | name |
"(" expr ")"
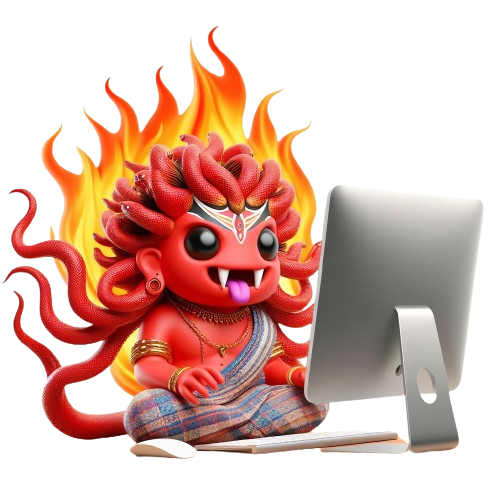
- Based on lox grammar and Python operator precedence.
Useful utils
# Annotations are used to define types of functions or structures
# Currently only @device, @native and @generic annotations are available.
# Annotations may have a single string parameter.
annotation: "@" name ( "(" (string | triple_quote_string ) ")" ) newline
parameters: parameter ("," parameter)*
parameter: name ":" datatype
st_end: newline | eof
newline: "\n" | "\r\n"
eof: "end of file"
ba_indent: "increase in indentation"
ba_dedent: "decrease is indentation"
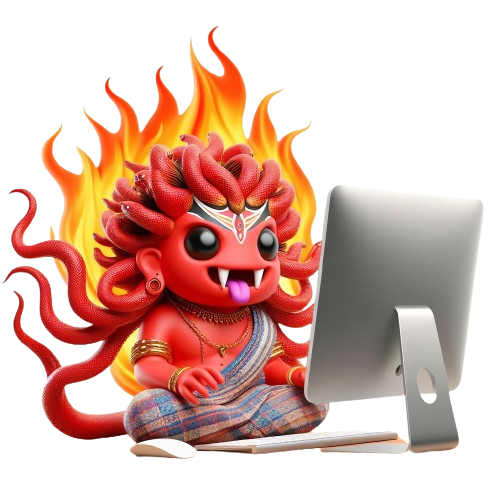
ba_indent
andba_dedent
gets calculated before parsing inblock_analyzer
phase.